A Quick And Thorough Guide On Javascript Query Selectors
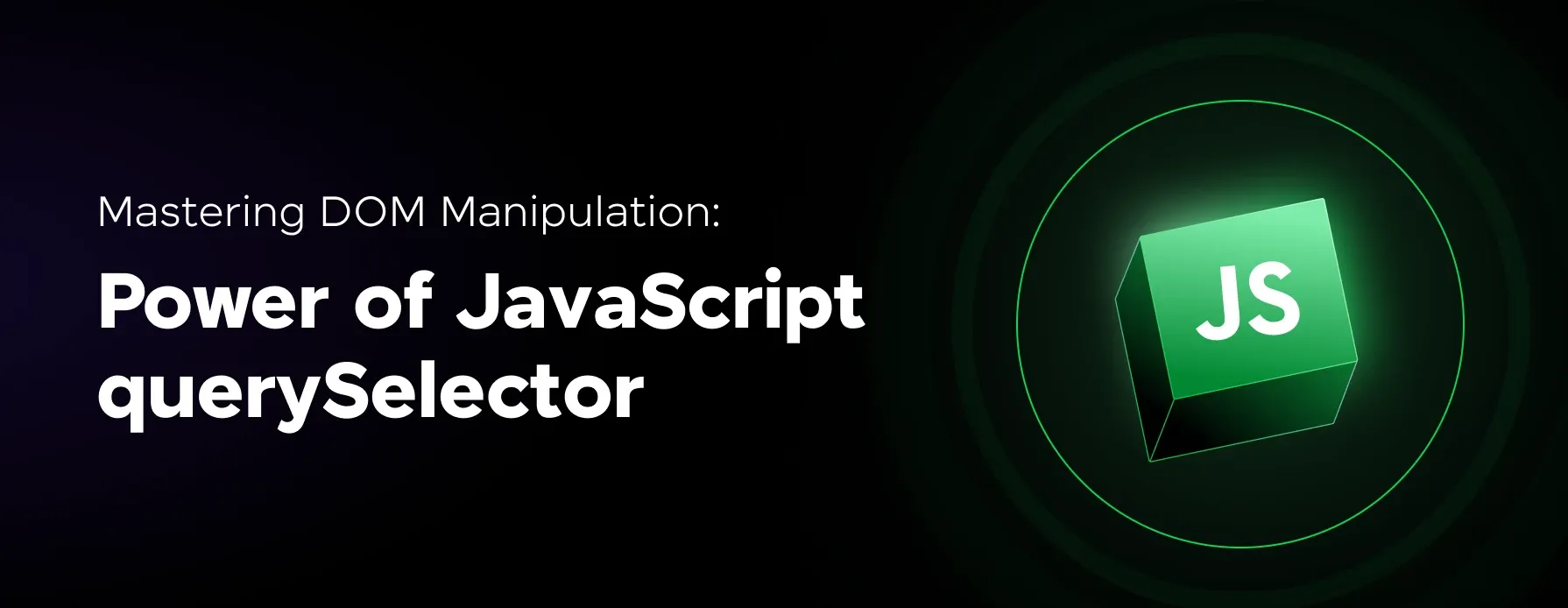
Having to go back and forth between writing backend and frontend code, I sometimes find myself needing a quick reference of the more advanced Javascript query selectors.
Javascript Query Selectors Worth Remembering
This is as much a reference for myself as it is for anyone else who's interested. I'll be including formal examples for each family of selectors, a brief description, and how they can be used.
Let's get started...
Core Methods
The main methods for querying elements: querySelector (for a single element) and querySelectorAll (for all matching elements). These are essential methods to interact with elements based on CSS-style selectors.
document.querySelector(selector)
: Returns the first element that matches the CSS selector.
document.querySelectorAll(selector)
: Returns all elements that match the CSS selector as a NodeList (similar to an array).
Basic Javascript Selectors
Simple selectors like universal (*), type (div), class (.class), and id (#id). These are the most common selectors for accessing elements directly by their type, class, or ID.
Formal Examples Include:
*
: Selects all elements
document.querySelectorAll('*')
element
: Selects all elements of the specified type
document.querySelectorAll('div')
.class
: Selects all elements with the specified class.
document.querySelectorAll('.my-class')
#id
: Selects the element with the specified id
document.querySelector('#my-id')
Basic And Advanced HTML Attribute Selectors
These are more advanced selectors that allow you to select elements based on their attributes (like type, id, or href) and support more specific queries, such as:
Exact matches: =
Starting with: ^=
Ending with: $=
Containing: *=
Whole word: ~=
Starting with or followed by a hyphen: |=
These are useful for dynamic content or complex conditions based on element attributes.
Formal Examples Include:
[attribute]
: Selects elements with the specified attribute
document.querySelectorAll('[type]')
[attribute="value"]
: Selects elements with an exact attribute match
document.querySelectorAll('[type="submit"]')
[attribute^="value"]
: Selects elements with an attribute starting with the specified value
document.querySelectorAll('[id^="Details-"]')
[attribute$="value"]
: Selects elements with an attribute ending with the specified value
document.querySelectorAll('[href$=".pdf"]')
[attribute*="value"]
: Selects elements with an attribute that contains the specified value anywhere in the string
document.querySelectorAll('[class*="button"]')
[attribute~="value"]
: Selects elements with an attribute that contains the specified word (separated by spaces).
document.querySelectorAll('[class~="header"]')
[attribute|="value"]
: Selects elements with an attribute that starts with the value or is followed by a hyphen.
document.querySelectorAll('[lang|="en"]')
Advanced Query Selector Combinators
These specify relationships between HTML elements. According to MDN, several relationships exist, such as:
Descendant: (A B)
Any B inside A
Child: (A > B)
Direct child B of A
Adjacent Sibling: (A + B)
The first B immediately following A
General Sibling: (A ~ B)
All B siblings after A
These Combinators help in narrowing down elements based on their relationship in the DOM tree.
Formal Examples Include:
A, B
: Selects all elements matching either selector A or B.
document.querySelectorAll('div, p')
A B
: Selects all elements B inside elements A (descendant selector)
document.querySelectorAll('ul li')
A > B
: Selects all elements B where B is a direct child of A.
document.querySelectorAll('div > p')
A + B
: Selects the immediate sibling element B that comes right after A
document.querySelectorAll('h1 + p')
A ~ B
: Selects all siblings B that follow after A
document.querySelectorAll('h2 ~ p')
Advanced Pseudo-Class Query Selectors
These selectors target HTML elements based on their state or position, such as:
Positional: (element
:first-child)
The first child of the targeted HTML element
Positional: (element
:nth-child(n))
The nth child of the targeted HTML element
Conditional: (element
:not(selector))
The first element where the related selector does not apply
These are commonly used to style or interact with elements based on their position, sibling order, or other dynamic conditions.
Formal Examples Include:
:first-child
: Selects the first child of its parent.
document.querySelectorAll('p:first-child')
:last-child
: Selects the last child of its parent
document.querySelectorAll('p:last-child')
:nth-child(n)
: Selects the nth child of its parent (1-based index)
document.querySelectorAll('li:nth-child(2)')
:nth-of-type(n)
: Selects the nth child of its type (e.g., nth <p>
element)
document.querySelectorAll('p:nth-of-type(3)')
:not(selector)
: Selects all elements that do not match the given selector.
document.querySelectorAll('div:not(.active)')
Invalid HTML Selectors
A couple valid CSS selectors that are valid but won't work when querying the DOM are:
::before
: Inserts content before the element.
::after
: Inserts content after the element
Conclusion
This quick guide can be used as a quick reference for JavaScript selectors, making it easy to find and use various selectors effectively in your code!
I thought to add related performance information but wanted to keep this article focused on one specific thing.
If you'd like to know more about query selector performance, please reach out.
See any typos or mistakes?? Please reach out